Sharing pre-request script among Postman collections
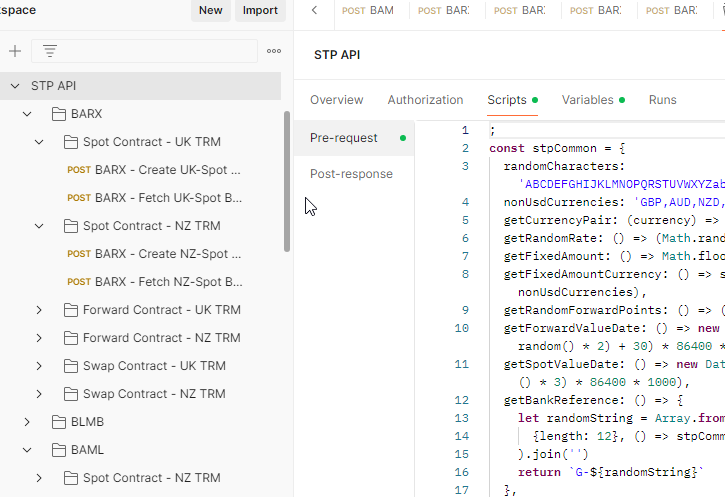
I tried a few ways to write a script that can be reused among a few of postman request collections and finally I got a solution I like.
- Using
const
orlet
keywords to define internal used objects or utility containers. For exampleconst stpCommon = { randomCharacters: 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'.split(''), nonUsdCurrencies: 'GBP,AUD,NZD,CAD,JPY,EUR'.split(','), getCurrencyPair: (currency) => `${currency}/USD`, getRandomRate: () => (Math.random() * 0.9 + 0.8).toFixed(5), getRandomElement: (array) => { if (array.length === 0) { throw new Error('Cannot get a random element from an empty array'); } const randomIndex = Math.floor(Math.random() * array.length); return array[randomIndex]; }, getNextWeekday: function (date) { const clonedDate = new Date(date); const day = clonedDate.getDay(); if (day === 6) { clonedDate.setDate(clonedDate.getDate() + 2); } else if (day === 0) { clonedDate.setDate(clonedDate.getDate() + 1); } }, getBasicRequest: () => { const fixedAmountCurrency = stpCommon.getFixedAmountCurrency() return { currencyPair: stpCommon.getCurrencyPair(fixedAmountCurrency), rate: stpCommon.getRandomRate(), side: "2", fixedAmount: stpCommon.getFixedAmount(), ... } ...
- Using object name itself in its member methods. don't use
this
keyword, see the last method in above example. - At the end of your script, using a conditional block to expose the object you want in Postman environment, normally one object is enough. For instance:
// the only way to expose an object in postman, is to define a object without any declaration keywords like var/let/const // the following is a hack to expose the object in postman without troubling Node.js as in Node.js, we are not allowed to // define a variable without any declaration keywords like var/let/const if (typeof(pm) !== 'undefined') { stpUtils = _stpUtils }
- At the end of your script, utilize conditional exports the objects that you want to use in Node.js environment, this step enables writing test cases for it. For example
const utilsA = {...} const utilsB = {...} const _utilsC = {something using utilsA & utilsB} // provided you want to use utilsC in postman if (typeof(pm) !== 'undefined') { utilsC = _utilsC } // and you want to expose all objects in your test cases if (typeof (module) !== 'undefined') { module.exports = { utilsA, utilsB, _utilsC } }
This way, you can paste all the script body into the postman scripts tab without trouble, while in Node.js env, you can freely use Jest or other unit test framework to write test cases for your shard library. What a great solution!