这里记录我的一周分享,通常在周六发布。
完颜兀术 每天跟chatgpt互相提供情绪价值,尽管GPT不需要,但我是个高尚的人
Isla I don't chase, I replace.
疗愈不是要你忘记过去,而是让你重新拥有未来。
戈城 agent不是用来解决一切问题的。人解决问题有几个层面:理解问题,干活,判断问题是否解决了。很多简单的或者贴近“理解”层面的事情不需要agent,而贴近“干活”的事情更需要agent,最后判断问题是否解决也不能靠agent。
如果你只是抱着试试看的心态,那么你只会以失败告终,你会一事无成。‘尝试’纯粹是一种借口,你还没有做,就已经给自己想好了退路。不能试验,你只有两个选择——做或者不做。
你的自信程度决定了你是否相信自己的能力,是否相信你自己。假如你根本不相信你能做到的话,那么你就根本不会动手去做,而假如你不开始去做,那么你就什么也得不到。
大量的创新都来自混搭,也就是说,它们只是把已经存在的事物又用新的方式表达了一遍。模仿、借鉴,甚至抄袭、山寨,在创新中都是司空见惯的。伟大的艺术家对此从不避讳。毕加索说过,"优秀的艺术家模仿,伟大的艺术家熏陶"。这句话深得乔布斯之心。苹果公司的初代iMac电脑因其全新的图形界面操作系统而为人称道,但这一创意却抄袭了施乐公司的发明。
成象化形面点师 好的人有想象不到的好,坏的人有想象不到的坏,各活各的,谁也无法改变谁;并且这些所谓“好”和“坏”的特质,其实又可以在不同的时刻、不同的境遇中统一在一个人身上。黑与白,阴与阳,不是东风压倒西风就是西风压倒东风,永远不会有一方真正消失,也不会有一方取得完全的“胜利”。对于许多社会事件,我觉得拿来作为鼓吹或批判时代地区大环境的材料都只能体现个人感情,并不能代表其真实的模样。重要的是,在明知没有胜负可分,即永远无法彻底“得胜”的前提下,我自己还是要选择成为其中我认为好的那一方。
Marskay (读了)吴晓乐《你的孩子不是你的孩子》, 4.3星。作者从多年家教经历中选讲了9个成绩或好或坏的孩子以及他们背后家长们的各种离奇古怪极端且偏执的教育养育方式,把小孩的幼小身心折磨得如入地狱,太触目惊心了。可惜虎妈鸡娃的家长们不会看这本书,看了也有一千万个理由反驳。但我们看了,我们很难过。
这世间最可怕的伤害,打的旗号叫“为你好”。
我也喜欢这个slogon: Today is better because I reached out.
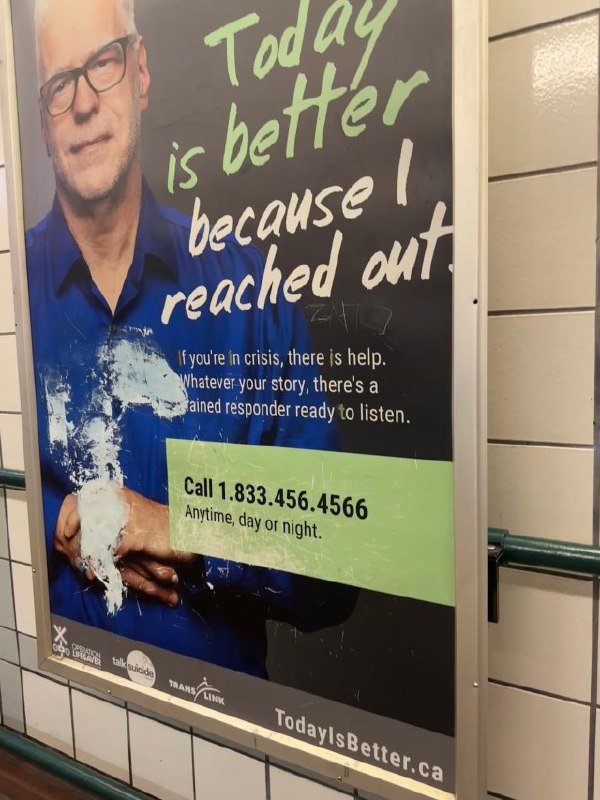
(图为加拿大自杀危机干预热线的广告)
Tuilindo 世界观需要一个人靠自己的历练获得,别人帮不了。
#想看 小名儿《白日梦想家》和《三块广告牌》都算是近年来对我的有些认知走向产生了某种影响的影片。
南美日记 坐在大巴上眼睛看着窗外阳光明媚的安第斯山脉风景,很美。太阳晒不到我,但能晒到靠窗的邻座大姐。她在伸手拉遮光窗帘之前先示意问我,看我点头了才把窗帘拉上,遮住她脸上的阳光。
高海拔地区的阳光很晒,就我的皮肤而言无遮挡多晒几分钟(半小时以内)就能晒伤。我邻座的本地大姐,她瞥见我在看风景,要拉上窗帘遮挡自己脸上的烈日之前,还先征得了我的同意。
我在这住了将近要两年了,还是会被本地人的细心与贴心程度震撼到。但我已经明白了,这种程度的温柔也只能在这样的社会中存在——若是在别的自私自利之人占主导的社会,要是偏有人觉得自己看风景的重要性高于邻座防晒的重要性,那这敏感的特质无疑会为敏感人自己带来无数灾难。
我之前所处的社会里,敏感是一种负担。但我现在所处的社会里,敏感是一种骄傲。敏感意味着你可以体谅所有人,那些人在不同的时刻也同样地体谅你。敏感意味着时常发现别人的照顾,敏感意味着生活中心怀善意与感激。在敏感是骄傲的社会里,所有人都能尽情地释放与接收所有人的关爱。
听书与阅读相比最大的区别与弊端,就是听书时人们是无法进行深度的思考的。当人们聚精会神地进行阅读时,读到自己之前从未见过的新观点时,读到一系列相对复杂的论述过程时,或者读到一些让自己感到眼前一亮的修辞时,可以反复阅读,或者停下来思考,从而加深对这些内容的理解与记忆。只有通过这样的过程,人们才能真正地消化和掌握有一定难度的新知识和新见解。
共勉。“1.要无条件自信,即使在做错的时候;2.不要想太多,定时清除消极思想;3.学会忘记痛苦,为阳光记忆腾出空间;4.敢于尝试,敢于丢脸;5.每天都是新的,烦恼痛苦不过夜;6.做人最高境界不是一味低调,也不是一味张扬,而是不卑不亢。
Will you fight less here?
Of course we will.
But if we should shout at each other now and then,
I want you to look up
and imagine how tiny our voices are out there.
So tiny, you can’t even hear them.
Look up at the stars, Jean-Luc.
Look up.
西瓦 “到21世纪第二个10年初期,原本以人与人之间的连接为主要功能的社交“网络”系统,转变成了以追求公众认可为主要目的的社交“媒体”平台,这些平台鼓励用户进行一对多的公开表演,以获取来自朋友乃至陌生人的认同和肯定,即使是不经常发布内容的用户,也受到这些应用程序设计的激励机制的影响。”
对不愉快经历的感知(今天天塌下来大的事情一年后再看也没有什么,请千万莫因一时挫折而自毁)

我们生命中最美好的时刻,并非是那些接受给予、放松享受的时刻,而是那些为了完成一件困难而有价值的事情,自愿将身心发挥到极限的时刻。
-- 米哈里·契克森米哈赖,他是"心流"(flow)概念的提出者
使我们感觉好些的不一定对我们好;使我们感到痛的不一定对我们不好。
Yingyu 做人要好似茶壶咁样,最紧要乐观,屁股都俾烧红了,仲有心情吹口哨…
(做人要像茶壶那样,最重要的是要乐观,即使屁股都要烧红了,照样有心情吹口哨)注意:普通话版可能有错讹,因为是我自己翻译的。
Scott Stillman
Forest = for rest
ask for more. the universe isn't on a budget
i love places that remind you how small you and your problems are
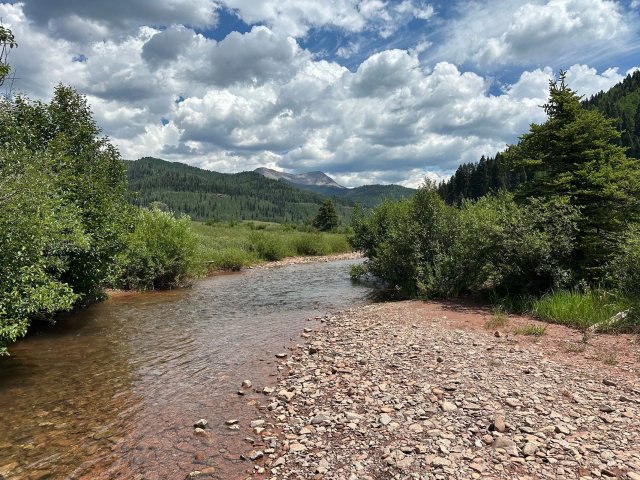